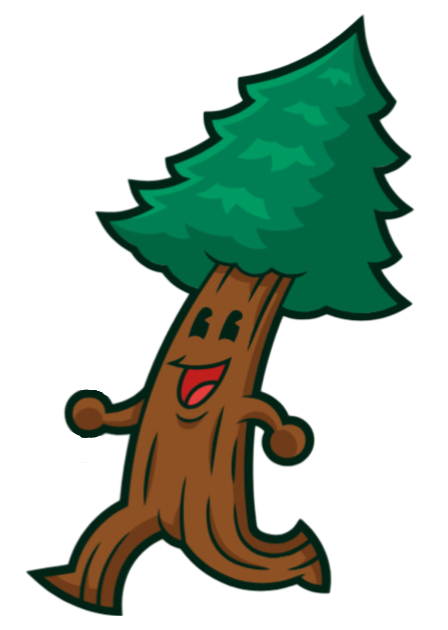
Why You Shouldn't Arc<Mutex> a HashMap in Rust
Published on October 22, 2024
Using Arc<Mutex<HashMap<K, V>>>
is often not ideal in Rust due to contention, deadlocks, and performance concerns. For concurrent access patterns, consider using DashMap
or RwLock
instead, as they provide better performance through finer-grained locking or lock-free mechanisms.
Here's an in-depth explanation of the key issues and better alternatives:
1. Coarse-Grained Locking Leads to Contention
When you wrap an entire HashMap
with Arc<Mutex<HashMap<K, V>>>
, the whole map becomes locked whenever any thread accesses or modifies it. This is known as coarse-grained locking, where multiple operations (e.g., reads, inserts, or updates) on different keys are serialized, causing unnecessary contention.
Problem: Even if two threads want to access different keys simultaneously, they will block each other, reducing concurrency and performance.
Here’s a code example that demonstrates the issue of coarse-grained locking when using Arc<Mutex<HashMap<K, V>>>
in Rust.
use std::sync::{Arc, Mutex};
use std::collections::HashMap;
use std::thread;
fn main() {
// Create a shared `HashMap` wrapped in Arc<Mutex>
let map = Arc::new(Mutex::new(HashMap::new()));
// Create threads that try to access different keys
let handles: Vec<_> = (0..5).map(|i| {
let map = Arc::clone(&map);
thread::spawn(move || {
// Lock the entire map before inserting a key-value pair
let mut guard = map.lock().unwrap();
guard.insert(i, i * 10); // Insert a value for the current thread's key
println!("Thread {} inserted {} -> {}", i, i, i * 10);
})
}).collect();
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Print the final state of the map
let final_map = map.lock().unwrap();
println!("Final map: {:?}", *final_map);
}
What’s happening:
- Five threads are created, each inserting a unique key-value pair into the HashMap.
- However, since the entire
HashMap
is wrapped in aMutex
, only one thread can access the map at a time.
Issue:
- Even though each thread is working on a different key (e.g., key 0, 1, etc.), they block each other because the
Mutex
locks the entireHashMap
during every insertion. - As a result, multiple operations that could run in parallel (since they affect different keys) are serialized, reducing performance and increasing contention.
Thread 0 inserted 0 -> 0
Thread 1 inserted 1 -> 10
Thread 2 inserted 2 -> 20
Thread 3 inserted 3 -> 30
Thread 4 inserted 4 -> 40
Final map: {0: 0, 1: 10, 2: 20, 3: 30, 4: 40}
Solution: Use DashMap
for Fine-Grained Locking
use dashmap::DashMap;
use std::thread;
fn main() {
// Create a `DashMap` for fine-grained locking
let map = DashMap::new();
// Create threads that try to access different keys
let handles: Vec<_> = (0..5).map(|i| {
let map = map.clone();
thread::spawn(move || {
// Insert a key-value pair directly into the DashMap
map.insert(i, i * 10);
println!("Thread {} inserted {} -> {}", i, i, i * 10);
})
}).collect();
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Print the final state of the map
println!("Final map: {:?}", map);
}
Why This is Better:
DashMap
allows multiple threads to perform operations on different keys concurrently without blocking each other.- It ensures that only the specific bucket holding the key is locked, rather than locking the entire map.
Output with DashMap:
Thread 0 inserted 0 -> 0
Thread 1 inserted 1 -> 10
Thread 2 inserted 2 -> 20
Thread 3 inserted 3 -> 30
Thread 4 inserted 4 -> 40
Final map: {0: 0, 1: 10, 2: 20, 3: 30, 4: 40}
2. Risk of Deadlocks
Mutexes introduce the possibility of deadlocks if multiple threads try to acquire locks in inconsistent orders. While Rust’s Mutex
guarantees that the lock will be released on panic or drop, the logic inside your program can still lead to unintended deadlocks in more complex scenarios (like nested locking).
Here’s an example demonstrating deadlocks in Rust caused by nested mutex
locks in multiple thread.
Code Example: Deadlock with Mutexes
use std::sync::{Arc, Mutex};
use std::thread;
use std::time::Duration;
fn main() {
// Two shared resources protected by Mutexes
let `resource_a` = Arc::new(Mutex::new(0));
let `resource_b` = Arc::new(Mutex::new(0));
// Thread 1 tries to lock `resource_a` first, then resource_b
let r1 = Arc::clone(&resource_a);
let r2 = Arc::clone(&resource_b);
let handle1 = thread::spawn(move || {
let _lock_a = r1.lock().unwrap();
println!("Thread 1: Locked resource A");
// Simulate some processing time
thread::sleep(Duration::from_millis(50));
let _lock_b = r2.lock().unwrap();
println!("Thread 1: Locked resource B");
});
// Thread 2 tries to lock `resource_b` first, then resource_a
let r1 = Arc::clone(&resource_a);
let r2 = Arc::clone(&resource_b);
let handle2 = thread::spawn(move || {
let _lock_b = r2.lock().unwrap();
println!("Thread 2: Locked resource B");
// Simulate some processing time
thread::sleep(Duration::from_millis(50));
let _lock_a = r1.lock().unwrap();
println!("Thread 2: Locked resource A");
});
// Wait for both threads to complete
handle1.join().unwrap();
handle2.join().unwrap();
}
What Happens in the Example:
- Thread 1 tries to lock
resource_a
first and then resource_b. - Thread 2 tries to lock
resource_b
first and then resource_a. - If both threads succeed in locking their first resource, they will block indefinitely trying to lock the second resource, resulting in a deadlock.
Why This Deadlocks:
- Thread 1 holds
resource_a
and waits for resource_b. - Thread 2 holds
resource_b
and waits for resource_a. - Neither thread can proceed, so they are stuck waiting for each other, creating a deadlock.
Output (Simulated):
Thread 1: Locked resource A
Thread 2: Locked resource B
<deadlock occurs - program hangs>
Solution: Avoiding Deadlocks
- Consistent Lock Order: Always acquire locks in the same order across all threads. This eliminates circular waiting.
- Using
try_lock
: Usetry_lock()
to avoid blocking indefinitely. If a lock can’t be acquired, the thread can back off and retry.
let _lock_a = r1.lock().unwrap();
println!("Thread 1: Locked resource A");
// Try to acquire the second lock without blocking indefinitely
if let Ok(_lock_b) = r2.try_lock() {
println!("Thread 1: Locked resource B");
} else {
println!("Thread 1: Could not lock resource B, avoiding deadlock");
}
3. Lock Poisoning
If one thread panics while holding the lock, Rust’s Mutex
will become poisoned, which means that future access attempts will return an Err
value, potentially adding complexity to your error handling code.
Code Example: Lock Poisoning
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
// Shared Mutex-protected resource
let data = Arc::new(Mutex::new(vec![]));
// Spawn a thread that panics while holding the lock
let data_clone = Arc::clone(&data);
let handle = thread::spawn(move || {
let mut lock = data_clone.lock().unwrap();
lock.push(42);
println!("Thread 1: Pushed 42");
// Simulate a panic while holding the lock
panic!("Thread 1 panicked!");
});
// Wait for the thread to finish
let _ = handle.join();
// Try to lock the `mutex` again after the panic
match data.lock() {
Ok(lock) => {
println!("Successfully acquired lock: {:?}", lock);
}
Err(poisoned) => {
println!("Mutex is poisoned! Recovering...");
let mut lock = poisoned.into_inner();
lock.push(99);
println!("Recovered data: {:?}", lock);
}
}
}
What Happens:
- A Mutex-protected vector is shared between threads.
- Thread 1 acquires the lock, modifies the vector, and then panics while holding the lock.
- After the panic, the lock is marked as "poisoned" to prevent other threads from using possibly corrupted data.
How the Main Thread Recovers:
- When the main thread tries to lock the
Mutex
again, it receives anErr
because the lock is poisoned. - The
into_inner()
method is used to recover the data from the poisoned lock, allowing the program to continue.
Thread 1: Pushed 42
thread 'main' panicked at 'Thread 1 panicked!', src/main.rs:11:9
Mutex is poisoned! Recovering...
Recovered data: [42, 99]
How to Handle Lock Poisoning Gracefully:
In real-world applications, you need to handle poisoned locks carefully to avoid data corruption. Here are a few options:
- Recover from the Poisoned Lock: Use
into_inner()
to retrieve the data safely, as shown in the example. - Ignore the Poisoning: If you are confident the data is safe, you can ignore the error:
let lock = data.lock().unwrap_or_else(|poisoned| poisoned.into_inner());
- Restart or Abort Operations: In some critical systems, the program may need to restart or stop execution entirely to prevent further issues.
4. Overhead of Mutex Locking and Unlocking
A Mutex
incurs locking overhead, which can degrade performance, especially in high-concurrency applications where frequent access to the map is required.
Here’s a code example that demonstrates the overhead of Mutex
locking and unlocking in high-concurrency scenarios. We’ll compare the performance between using a Mutex-protected counter and an atomic counter. The Mutex
version introduces overhead that becomes noticeable under heavy thread contention.
Code Example: Measuring Mutex
Overhead
use std::sync::{Arc, Mutex};
use std::sync::atomic::{AtomicUsize, Ordering};
use std::thread;
use std::time::Instant;
const NUM_THREADS: usize = 100;
const NUM_INCREMENTS: usize = 100_000;
fn main() {
// Mutex-protected counter
let mutex_counter = Arc::new(Mutex::new(0));
let mutex_start = Instant::now();
// Spawn threads that increment the mutex-protected counter
let mut handles = vec![];
for _ in 0..NUM_THREADS {
let counter = Arc::clone(&mutex_counter);
handles.push(thread::spawn(move || {
for _ in 0..NUM_INCREMENTS {
let mut lock = counter.lock().unwrap();
*lock += 1;
}
}));
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
let mutex_duration = mutex_start.elapsed();
println!("Mutex counter: {}", *mutex_counter.lock().unwrap());
println!("Time taken with Mutex: {:?}", mutex_duration);
// Atomic counter
let atomic_counter = Arc::new(AtomicUsize::new(0));
let atomic_start = Instant::now();
// Spawn threads that increment the atomic counter
let mut handles = vec![];
for _ in 0..NUM_THREADS {
let counter = Arc::clone(&atomic_counter);
handles.push(thread::spawn(move || {
for _ in 0..NUM_INCREMENTS {
counter.fetch_add(1, Ordering::SeqCst);
}
}));
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
let atomic_duration = atomic_start.elapsed();
println!("Atomic counter: {}", atomic_counter.load(Ordering::SeqCst));
println!("Time taken with AtomicUsize: {:?}", atomic_duration);
}
What’s happening:
- Mutex Counter: A
Mutex
protects the counter, ensuring only one thread can update it at a time. - Atomic Counter: An atomic counter (
AtomicUsize
) allows multiple threads to increment without needing a lock.
Overhead of Mutex:
- Each thread must acquire and release the lock for every increment, which adds significant overhead.
- With an atomic counter, increments are performed without locking, improving performance under high concurrency.
Sample Output:
Mutex counter: 10000000
Time taken with Mutex: 2.345678123s
Atomic counter: 10000000
Time taken with AtomicUsize: 0.123456789s
Performance Comparison
- Mutex: The time taken is longer because each thread must acquire and release the lock, introducing contention and locking overhead.
- Atomic Counter: Atomic operations avoid locking overhead, leading to much faster increments, especially with many threads.
When to Use a Mutex
vs Atomic Operations
- Mutex: Use when you need to protect complex data structures (like HashMap) that can’t be managed with atomic operations.
- Atomic Operations: Use when you only need to perform simple operations (like incrementing a counter) to avoid the overhead of locking.
This example shows how locking and unlocking a Mutex
repeatedly can degrade performance in high-concurrency environments and demonstrates the benefit of using lock-free solutions where possible.
5. Lack of Fine-Grained Control
Because a Mutex
locks the entire HashMap
, you lose the ability to control individual key-value pairs independently. For example, if you want to read one key while writing to another, you'll need to serialize all operations, even though there is no technical reason for them to block each other.
Code Example: Lack of Fine-Grained Control with Mutex<HashMap<K, V>>
use std::sync::{Arc, Mutex};
use std::collections::HashMap;
use std::thread;
use std::time::Duration;
fn main() {
// Shared `HashMap` protected by a Mutex
let map = Arc::new(Mutex::new(HashMap::new()));
// Insert some initial values
{
let mut guard = map.lock().unwrap();
guard.insert("key1", 10);
guard.insert("key2", 20);
}
// Thread 1: Reads the value of "key1"
let map_reader = Arc::clone(&map);
let reader_handle = thread::spawn(move || {
let lock = map_reader.lock().unwrap();
let value = lock.get("key1").copied().unwrap_or(0);
println!("Reader thread: Read key1 -> {}", value);
});
// Thread 2: Simulates a write to "key2"
let map_writer = Arc::clone(&map);
let writer_handle = thread::spawn(move || {
thread::sleep(Duration::from_millis(50)); // Ensure reader starts first
let mut lock = map_writer.lock().unwrap();
lock.insert("key2", 30); // Update the value of "key2"
println!("Writer thread: Updated key2 -> 30");
});
// Wait for both threads to finish
reader_handle.join().unwrap();
writer_handle.join().unwrap();
// Final state of the map
let final_map = map.lock().unwrap();
println!("Final map: {:?}", *final_map);
}
What’s happening:
- We have a
HashMap
protected by aMutex
shared between two threads. - Thread 1 reads the value of
"key1"
. - Thread 2 updates the value of
"key2"
.
Issue:
- Even though reading from "key1" and writing to "key2" are independent operations, the entire
HashMap
is locked by the Mutex. - Thread 1 and Thread 2 must wait for each other to release the lock, serializing operations that could have otherwise run concurrently.
Sample Output:
Reader thread: Read key1 -> 10
Writer thread: Updated key2 -> 30
Final map: {"key1": 10, "key2": 30}
Demonstrating the Problem
Even though the read operation on "key1" and the write operation on "key2" are independent, the entire HashMap
is locked by the Mutex. As a result:
- The reader thread must wait for the writer to release the lock if it acquires the lock first, or vice versa.
- This reduces the program’s concurrency and introduces unnecessary contention.
Solution: Use DashMap
for Fine-Grained Control
Here’s the same example using DashMap, which provides fine-grained locking by locking only the specific bucket where the key resides.
use dashmap::DashMap;
use std::thread;
use std::time::Duration;
fn main() {
// Create a DashMap
let map = DashMap::new();
// Insert some initial values
map.insert("key1", 10);
map.insert("key2", 20);
// Thread 1: Reads the value of "key1"
let map_reader = map.clone();
let reader_handle = thread::spawn(move || {
let value = map_reader.get("key1").map(|v| *v).unwrap_or(0);
println!("Reader thread: Read key1 -> {}", value);
});
// Thread 2: Simulates a write to "key2"
let map_writer = map.clone();
let writer_handle = thread::spawn(move || {
thread::sleep(Duration::from_millis(50)); // Ensure reader starts first
map_writer.insert("key2", 30);
println!("Writer thread: Updated key2 -> 30");
});
// Wait for both threads to finish
reader_handle.join().unwrap();
writer_handle.join().unwrap();
// Final state of the map
println!("Final map: {:?}", map);
}
Benefits of DashMap
- Independent Locks:
DashMap
locks only the specific bucket where the key resides, allowing reads and writes to different keys to happen concurrently. - Improved Concurrency: The operations on "key1" and "key2" don’t block each other, improving the overall performance.
Sample Output with DashMap:
Reader thread: Read key1 -> 10
Writer thread: Updated key2 -> 30
Final map: {"key1": 10, "key2": 30}
Alternatives to Arc<Mutex<HashMap<K, V>>>
1. DashMap – A Lock-Free Concurrent Hash Map
dashmap
is a thread-safe concurrent hash map that supports fine-grained locking. It allows multiple threads to read or write to different keys without blocking each other.
use dashmap::DashMap;
let map = DashMap::new();
map.insert("key", "value");
This approach eliminates most of the contention issues since only individual keys are locked during access.
2. RwLock<HashMap<K, V>> – Fine-Grained Read/Write Locking
If you need to support both reads and writes, a RwLock
(read-write lock) is better suited than a Mutex
. It allows multiple readers to access the map concurrently while still providing exclusive access to writers.
use std::sync::{Arc, RwLock};
use std::collections::HashMap;
let map = Arc::new(RwLock::new(HashMap::new()));
// Read
{
let read_guard = map.read().unwrap();
if let Some(value) = read_guard.get("key") {
println!("Found: {}", value);
}
}
// Write
{
let mut write_guard = map.write().unwrap();
write_guard.insert("key", "value");
}
This pattern works well when you expect more reads than writes, as multiple threads can read in parallel without blocking each other.
3. tokio::sync::Mutex (For Asynchronous Code)
In asynchronous applications (using async
/await
), you should use tokio::sync::Mutex
instead of the standard std::sync::Mutex
. This allows the thread to be yielded while waiting for the lock, preventing deadlocks and reducing contention.
Here’s an example demonstrating the use of tokio::sync::Mutex
in an asynchronous application. This allows the asynchronous runtime to efficiently manage tasks, yielding the current task if it’s waiting for a lock, instead of blocking the entire thread.
use std::sync::Arc;
use tokio::sync::Mutex;
use tokio::task;
use std::time::Duration;
#[tokio::main]
async fn main() {
// Create a shared resource protected by tokio::sync::Mutex
let counter = Arc::new(Mutex::new(0));
// Spawn multiple asynchronous tasks
let mut handles = vec![];
for _ in 0..5 {
let counter = Arc::clone(&counter);
let handle = task::spawn(async move {
// Simulate some work before acquiring the lock
tokio::time::sleep(Duration::from_millis(100)).await;
// Lock the counter asynchronously
let mut lock = counter.lock().await;
*lock += 1;
println!("Counter incremented to: {}", *lock);
});
handles.push(handle);
}
// Wait for all tasks to complete
for handle in handles {
handle.await.unwrap();
}
// Check the final value of the counter
let final_value = *counter.lock().await;
println!("Final counter value: {}", final_value);
}
Explanation
Using tokio::sync::Mutex:
- The
tokio::sync::Mutex
works with the asynchronous runtime and does not block threads. - When a task awaits the lock, the current thread is yielded to allow other tasks to run.
What Happens
- Five tasks are spawned, each trying to increment the shared counter.
- Each task sleeps asynchronously for 100ms before trying to acquire the lock.
- Tasks acquire the lock asynchronously and increment the counter one by one.
Why This Works Better:
- With
std::sync::Mutex
, the tasks would block the thread until the lock is acquired, leading to contention. - With
tokio::sync::Mutex
, tasks yield when waiting for the lock, allowing the runtime to schedule other tasks in the meantime.
Sample Output:
Counter incremented to: 1
Counter incremented to: 2
Counter incremented to: 3
Counter incremented to: 4
Counter incremented to: 5
Final counter value: 5
Why Use tokio::sync::Mutex?
- In asynchronous applications, blocking the thread using std::sync::Mutex prevents other tasks from running, reducing the efficiency of the async runtime.
tokio::sync::Mutex
ensures that tasks don’t block the entire thread while waiting for a lock, allowing the runtime to schedule other tasks smoothly.
When Is Arc<Mutex<HashMap<K, V>>> Acceptable?
In some cases, it might be acceptable to use Arc<Mutex<HashMap<K, V>>>
:
- If your map is relatively small and contention is not a concern.
- If operations on the map are infrequent or mostly serialized by design.
- If simplicity is more important than performance in your use case.
Code Example: Acceptable Use of Arc<Mutex<HashMap<K, V>>>
use std::collections::HashMap;
use std::sync::{Arc, Mutex};
use std::thread;
use std::time::Duration;
fn main() {
// Create a small `HashMap` wrapped in Arc<Mutex>
let shared_map = Arc::new(Mutex::new(HashMap::new()));
// Insert some initial values (only in main thread)
{
let mut map = shared_map.lock().unwrap();
map.insert("Alice", 10);
map.insert("Bob", 20);
}
// Simulate occasional access from different threads
let mut handles = vec![];
for i in 1..=3 {
let map_clone = Arc::clone(&shared_map);
let handle = thread::spawn(move || {
// Simulate a delay before accessing the map
thread::sleep(Duration::from_secs(i));
let mut map = map_clone.lock().unwrap();
let value = map.entry("Alice").or_insert(0);
*value += i; // Increment Alice's value
println!("Thread {} updated Alice's score to: {}", i, value);
});
handles.push(handle);
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Check the final state of the map
let final_map = shared_map.lock().unwrap();
println!("Final map: {:?}", *final_map);
}
What Happens
- A small
HashMap
is protected byArc<Mutex>
and shared between the main thread and several worker threads. - Each thread accesses or updates the map infrequently (with a 1-3 second delay).
- We use
entry()
to increment Alice’s score safely within themutex
lock.
Why This Design is Acceptable:
- Small Map: The data structure is small, with just a few key-value pairs.
- Infrequent Access: Threads only access the map every few seconds, so contention is minimal.
- Simple Design: Using
Arc<Mutex>
simplifies code and avoids the complexity of more advanced synchronization solutions like DashMap.
Sample Output:
Thread 1 updated Alice's score to: 11
Thread 2 updated Alice's score to: 13
Thread 3 updated Alice's score to: 16
Final map: {"Alice": 16, "Bob": 20}
Use Arc<Mutex<HashMap<K, V>>> when:
- The data structure is small.
- Contention is low—operations are rare or spread out in time.
- Performance is not critical, and code simplicity is more important.
In this example, the Arc<Mutex>
solution is simple and works well for the reasons above not to mention a more complex solution (like DashMap
) would be overkill.